How to Use PowerShell Exit Codes for Scripting Automation
Step-by-step guide on leveraging PowerShell exit codes to automate script error handling and integrate with external automation tools.
When it comes to PowerShell scripting and automation, understanding exit codes (or return codes) is essential for effectively managing errors and troubleshooting. These exit codes serve as indicators, letting you know whether a script or command executed successfully or ran into issues.
In this article, we’ll dive into what PowerShell exit codes are, why they’re important and how to handle them like a pro.
What are PowerShell Exit Codes?
An exit code is a numeric value that a script, command or application returns when it finishes executing. PowerShell, similar to other scripting languages, uses these exit codes to signal the result of a process:
- 0: This means success (no errors).
- Non-zero values: These indicate failure or specific types of errors.
Exit codes are particularly valuable in automated settings like CI/CD pipelines, where they help determine the next steps based on whether tasks succeeded or failed.
Why are PowerShell Exit Codes Important?
- Error Identification: Exit codes give you insights into the types of problems encountered during execution.
- Automation Decisions: Scripts and tools can leverage exit codes to make conditional choices, like retrying a command or stopping execution altogether.
- Debugging: Developers can track down and resolve errors based on the exit codes returned by PowerShell scripts or external applications.
How to Use Exit Codes in PowerShell
1. Using the Exit
Command
The Exit command in PowerShell is your go-to for ending a script and specifying an exit code.
Example:
# Script.ps1
if (-Not (Test-Path "C:\\\\ImportantFile.txt")) {
Write-Host "File is not found!"
Exit 1 # This sets the exit code to 1, indicating it a failure
} else {
Write-Host "File is exists."
Exit 0 # This sets the exit code to 0, indicating it a success
}
2. Retrieving Exit Codes
You can easily grab the exit code from the last command you ran in PowerShell using the $LASTEXITCODE automatic variable.
Example:
# Execute a command
ping 127.0.0.1
# Now, check its exit code
Write-Host "Exit Code: $LASTEXITCODE"
3.Using Try-Catch for Error Handling
PowerShell’s structured error handling lets you tackle errors effectively and return the right exit codes.
Example:
try {
# Try to perform a risky operation
Get-Content "C:\\NonExistentFile.txt"
Exit 0
} catch {
Write-Host "An error occurred: $($_.Exception.Message)"
Exit 1
}
Common Exit Codes in PowerShell
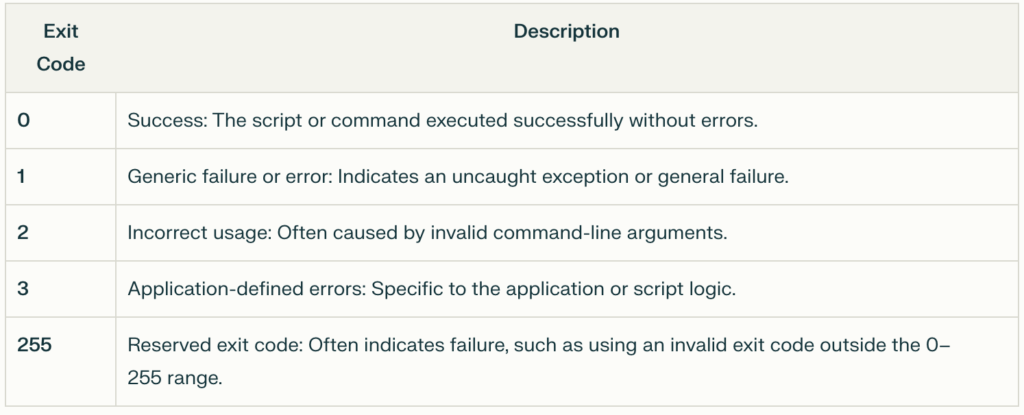
Tips for Managing Exit Codes
- Standardize Exit Codes: Stick to a consistent set of exit codes in your scripts. This makes them easier to read and maintain.
- Document Your Codes: Make sure to clearly explain what each exit code means in your script's documentation. It’ll save you and others a lot of confusion down the line.
- Test Your Scripts: Run through various scenarios to check that your scripts are handling and returning exit codes as they should.
- Monitor $ErrorActionPreference: This setting is key for controlling how PowerShell deals with errors on a global scale.
Conclusion
PowerShell exit codes are a powerful tool for managing errors and automating tasks. When both administrators and developers understand how to handle these codes effectively, they can streamline their workflows, enhance the reliability of their scripts and make troubleshooting much simpler. Whether you're working with basic scripts or complex automation pipelines, utilizing exit codes ensures everything runs smoothly and predictably.
Frequently asked questions:
-
How do exit codes differ from error messages in PowerShell?
Exit codes are numeric values representing the execution outcome, while error messages provide descriptive information about the issue.
-
Can I use custom exit codes in PowerShell scripts?
Yes, you can define and use custom exit codes to represent specific conditions or errors in your scripts.
-
What happens if I don’t specify an exit code in a script?
If you don’t use the Exit statement, PowerShell exits with a default code of 0, assuming the script executed successfully.
-
How do external applications influence $LASTEXITCODE?
When running external commands or executables from PowerShell, $LASTEXITCODE captures the exit code returned by those applications.
-
How can I suppress errors and prevent non-zero exit codes?
You can use the -ErrorAction SilentlyContinue parameter to suppress errors during command execution: "Get-Content "C:\NonExistentFile.txt" -ErrorAction SilentlyContinue"